HappyCoding/JavaScript
JS Function
ELLIE_ing
2022. 2. 20. 01:25
hoisting
정의되기 전에 함수 선언에 대한 액세스를 허용하는 JavaScript 의 기능
greetWorld(); // Output: Hello, World!
function greetWorld() {
console.log('Hello, World!');
}
//hoisting
catName("Tiger");
function catName(name) {
console.log("My cat's name is " + name);
}
/*
The result of the code above is: "My cat's name is Tiger"
*/
// hoisting없으면 동일한 코드를 작성해야함
function catName(name) {
console.log("My cat's name is " + name);
}
catName("Tiger");
/*
The result of the code above is the same: "My cat's name is Tiger"
*/
출처 : https://developer.mozilla.org/en-US/docs/Glossary/Hoisting
Function Declarations
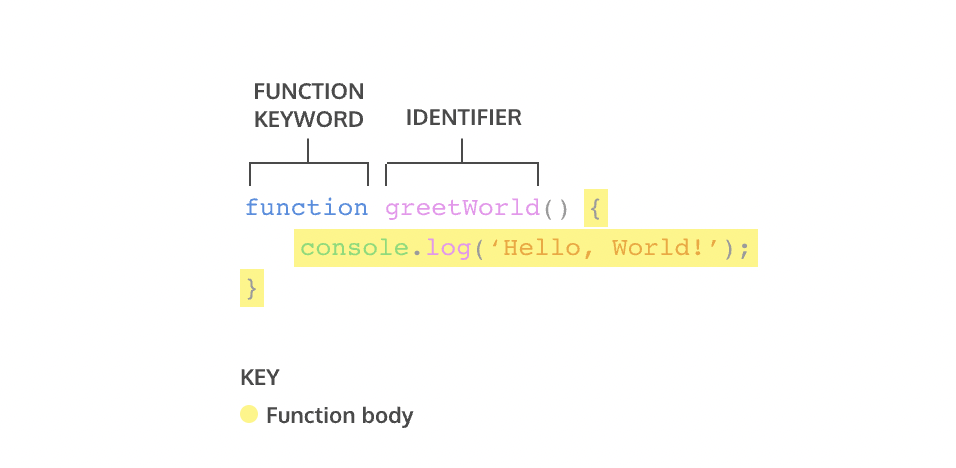
function getReminder(){
console.log('Water the plants');
};
function greetInSpanish(){
console.log('Buenas Tardes.');
}
Calling a Function
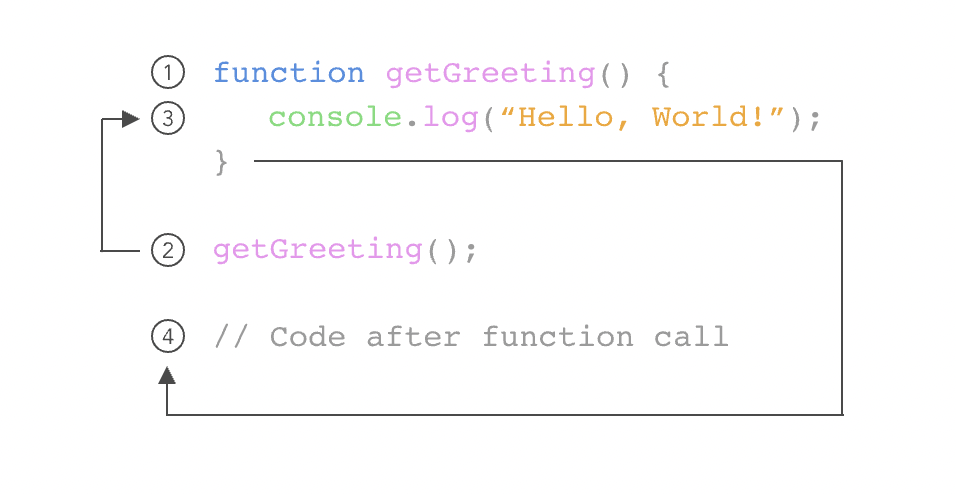
function sayThanks(){
console.log('Thank you for your purchase! We appreciate your business.');
}
sayThanks();
sayThanks();sayThanks();
Parameters and Arguments
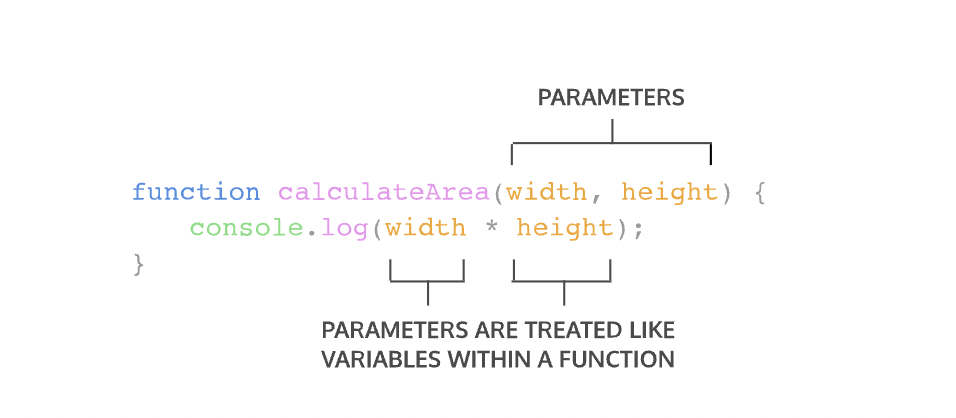
let name = 'Ellie';
function sayThanks(name) {
console.log('Thank you for your purchase '+name+'! We appreciate your business.');
}
sayThanks('Cole');
Default Parameters
function greeting (name = 'stranger') {
console.log(`Hello, ${name}!`)
}
greeting('Nick') // Output: Hello, Nick!
greeting() // Output: Hello, stranger!
function makeShoppingList(item1='milk', item2='bread', item3='eggs'){
console.log(`Remember to buy ${item1}`);
console.log(`Remember to buy ${item2}`);
console.log(`Remember to buy ${item3}`);
}
Return
function rectangleArea(width, height) {
let area = width * height;
}
console.log(rectangleArea(5, 7)) // Prints undefined
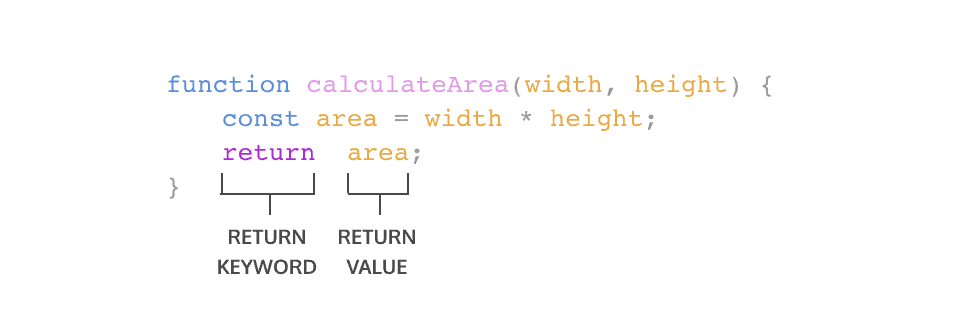
function rectangleArea(width, height) {
if (width < 0 || height < 0) {
return 'You need positive integers to calculate area!';
}
return width * height;
}
function monitorCount(rows, columns){
return rows * columns;
}
const numOfMonitors = monitorCount(5,4);
console.log(numOfMonitors);
Helper Functions
function multiplyByNineFifths(number) {
return number * (9/5);
};
function getFahrenheit(celsius) {
return multiplyByNineFifths(celsius) + 32;
};
getFahrenheit(15); // Returns 59
function monitorCount(rows, columns) {
return rows * columns;
}
function costOfMonitors(rows,columns){
return monitorCount(rows,columns)*200;
}
const totalCost = costOfMonitors(5,4);
console.log(totalCost);
Function Expressions
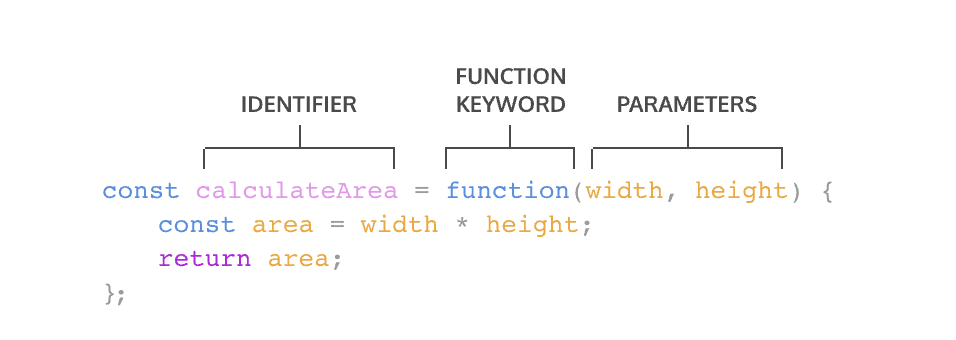
const plantNeedsWater = function(day){
if (day === 'Wednesday'){
return true;
} else{
return false;
plantNeedsWater('Tuesday');
}
};
console.log(plantNeedsWater('Tuesday'));
Arrow Functions
ES6 introduced arrow function syntax, a shorter way to write functions by using the special “fat arrow” () => notation.
const rectangleArea = (width, height) => {
let area = width * height;
return area;
};
if (day === 'Wednesday') {
return true;
} else {
return false;
}
};
Concise Body Arrow Functions
- Functions that take only a single parameter do not need that parameter to be enclosed in parentheses. However, if a function takes zero or multiple parameters, parentheses are required.
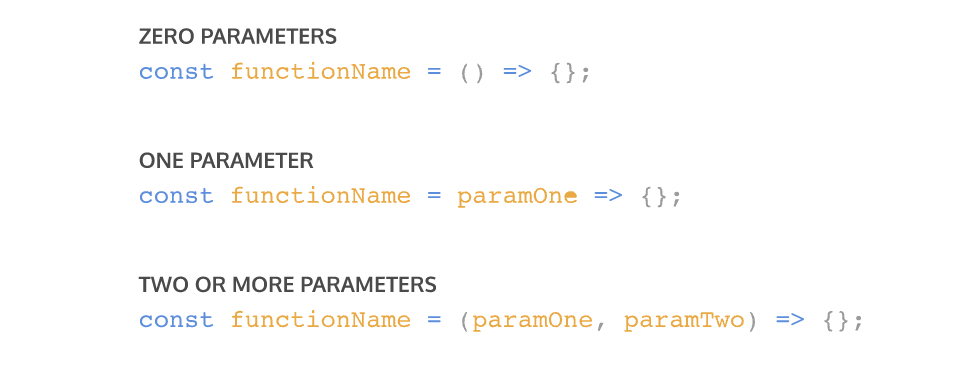
2.
A function body composed of a single-line block does not need curly braces. Without the curly braces, whatever that line evaluates will be automatically returned. The contents of the block should immediately follow the arrow => and the return keyword can be removed. This is referred to as implicit return.
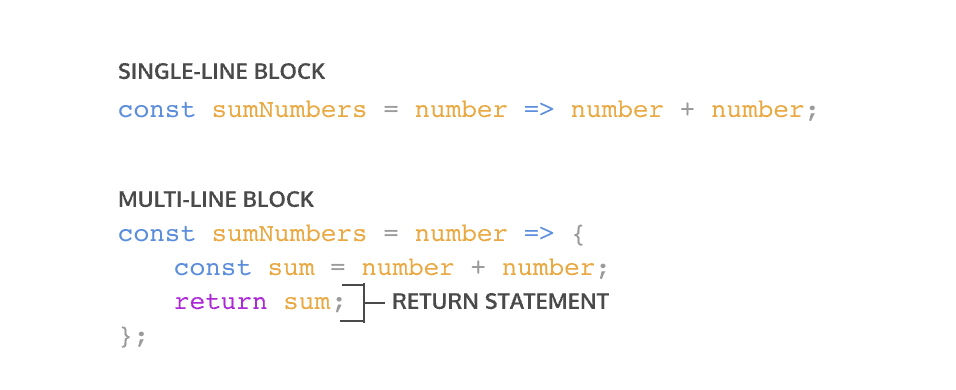
const squareNum = (num) => {
return num * num;
};
refactor->
const squareNum = num => num * num;
exercise
const plantNeedsWater = day => day === 'Wednesday' ;